EL은 기본 객체의 getter 메서드를 그대로 이용할 수 있습니다.
다만, 일반 표현식에서 사용하는 것 처럼 메서드 형태로 이용하기보다는
변수 이름으로 가져오는 방식을 주로 사용합니다.
자세한 사용 방법은 아래 예제를 통해 확인하실 수 있습니다.
request header에 접근하는 방법
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>How to get request header?</title>
</head>
<body>
<h3>헤더에 접근하는 방법</h3>
HOST : <%=request.getHeader("host") %><br>
CONNECTION : <%=request.getHeader("connection") %><br>
<h3>헤더에 접근하는 방법(EL)</h3>
HOST : ${header.host }<br>
CONNECTION : ${header.connection }<br>
</body>
</html>
결과 창
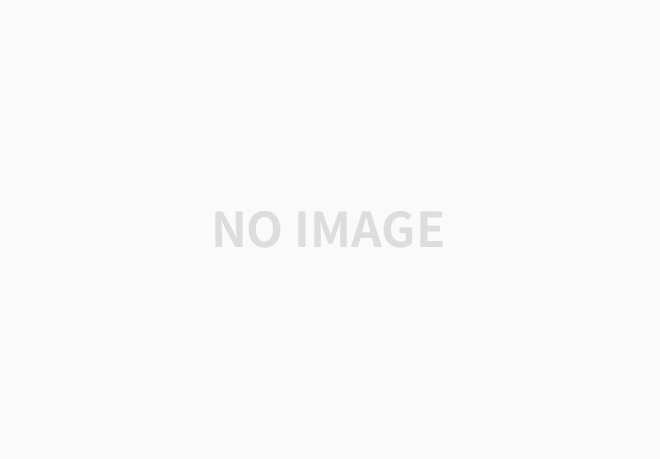
기본객체에 접근하는 방법
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>How to get request header?</title>
<style>
td{
border: 1px solid blue;
width: 200px;
}
*{
font-size: 14px;
font-family: 나눔고딕;
}
</style>
</head>
<body>
<table>
<tr>
<td>표현식</td>
<td>결과값</td>
<td>EL</td>
<td>결과값</td>
</tr>
<tr>
<td><\%=request.getContextPath() %></td>
<td><%=request.getContextPath() %></td>
<td>\${pageContext.request.contextPath }</td>
<td>${pageContext.request.contextPath }</td>
</tr>
<tr>
<td><\%=request.getRemoteAddr() %></td>
<td><%=request.getRemoteAddr() %></td>
<td>\${pageContext.request.remoteAddr }</td>
<td>${pageContext.request.remoteAddr }</td>
</tr>
<tr>
<td><\%=application.getRealPath("") %></td>
<td><%=application.getRealPath("") %></td>
<td>\${pageContext.servletContext.getRealPath('') }</td>
<td>${pageContext.servletContext.getRealPath('') }</td>
</tr>
</table>
</body>
</html>
결과 창
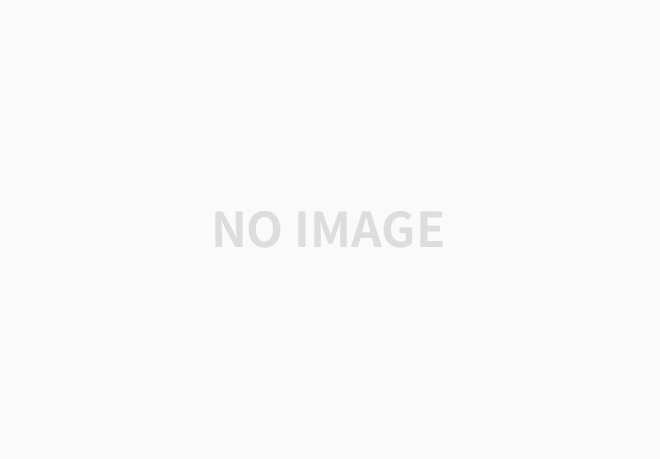
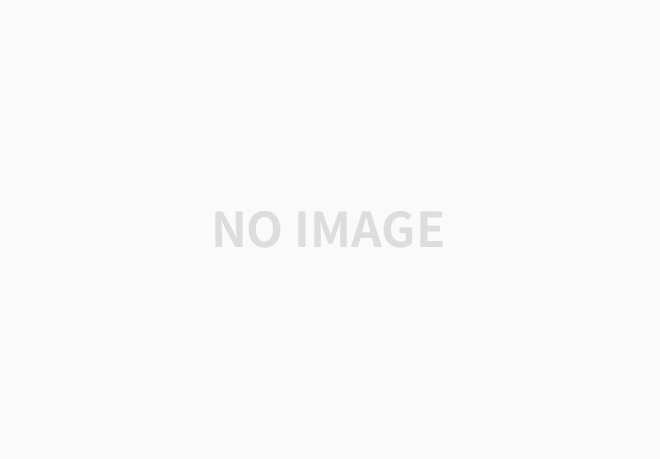
EL로 Scope에 접근하는 방법
EL로 Scope에 접근할 때에 주의사항은, Scope에 값이 없을 때 NullPointerException대신 ''(공백)을 반환한다는 것입니다.
기본적으로 EL로 Scope에 접근하는 형식은 다음과 같습니다.
접근할 때 앞쪽의 pageScope, request, session, application은 생략할 수 있습니다.
생략한 상태로 값을 접근할 때에는 pageScope -> request -> session -> application 순서로 값을 체크하게 됩니다.
// pageScope에 접근할 때
${pageScope.(저장한 값의 Key)}
// requestScope에 접근할 때
${request.(저장한 값의 Key)}
// sessionScope에 접근할 때
${session.(저장한 값의 Key)}
// applicationScope에 접근할 때
${application.(저장한 값의 Key)}
EL로 쿼리스트링 접근 방법
Ex URL : http://localhost/devEricServers/el/expressionLanguage.jsp?mem_id=a001&mem_pass=asdfasd
위 URL에는 GET형식으로 mem_id와 mem_pass의 값을 넘겨받을 수 있습니다.
EL에서는 위와 같은 파라미터 값을 'param'을 통해 다음과 같이 접근할 수 있습니다.
ID : ${param.mem_id}<br>
PASS : ${param.mem_pass}<br>
EL로 배열, 컬랙션 접근 방법
EL로 접근한 값이 배열인 경우 인덱스로 접근이 가능하고, Map일 경우에는 Key값을 통해 접근할 수 있습니다.
arr에서 0번째 인덱스 값 꺼내기 : ${arr[0]}<br>
myMap에서 myKey로 매핑된 값 꺼내기 : ${myMap['myKey']<br>
EL로 NULL 비교방법
EL에서는 null값을 일반 수식(value == null)을 통해서도 비교할 수 있지만
EL이 가지고 있는 'empty', 'not empty'를 주로 이용합니다.
NULL 비교 예제
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
pageContext.setAttribute("myValue","Hello Eric!");
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<style type="text/css">
td{
border : 1px solid blue;
font-size: 20px;
font-family: 나눔고딕;
width: 150px;
}
</style>
</head>
<body>
<table>
<tr>
<td>Key</td>
<td>Value</td>
<td>EL</td>
<td>is Null?</td>
<td>EL</td>
<td>is Not Null?</td>
</tr>
<tr>
<td>myValue</td>
<td>${myValue}</td>
<td>\${empty myValue}</td>
<td>${empty myValue}</td>
<td>\${not empty myValue}</td>
<td>${not empty myValue}</td>
</tr>
<tr>
<td>nullValue</td>
<td>${nullValue}</td>
<td>\${empty nullValue}</td>
<td>${empty nullValue}</td>
<td>\${not empty nullValue}</td>
<td>${not empty nullValue}</td>
</tr>
</table>
</body>
</html>
결과 창
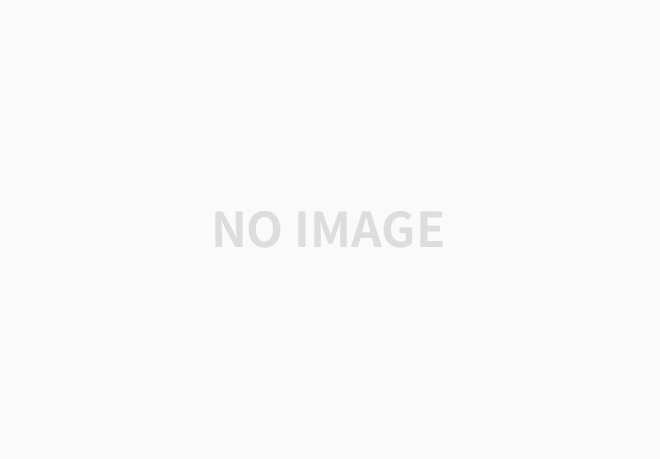
nullValue를 키로 넘긴 값은 NULL이기 때문에, 공백 문자가 온 것을 확인할 수 있습니다.
'Web > JSP' 카테고리의 다른 글
[JSP] JSTL Core - forEach, forTockens (0) | 2019.07.22 |
---|---|
[JSP] JSTL 설치 방법 (0) | 2019.07.22 |
[JSP] EL(Expression Language) 사칙연산, 논리연산 (0) | 2019.07.22 |
[JSP] remoteAddr()이 IPv6(0:0:0:0:0:0:0:1) 루프백으로 나올 경우 (0) | 2019.07.22 |
[JSP] Edwith 프로젝트 To-Do List (1) | 2019.07.17 |
댓글